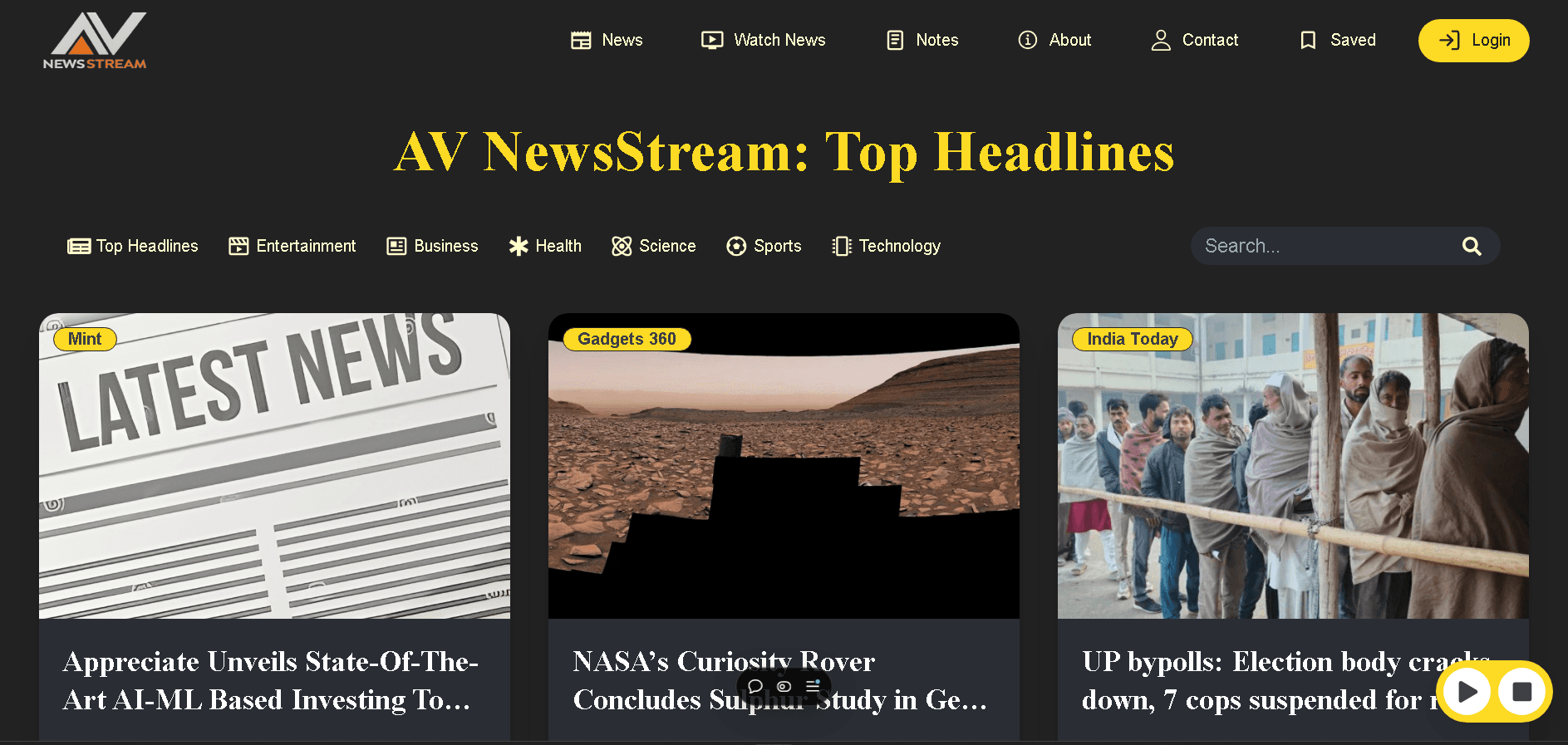
AV News Stream
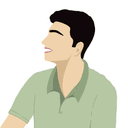
Aman Suryavanshi
Passionate web developer and designer with expertise in creating functional, user-centric digital experiences using modern technologies like React, Tailwind CSS, and JavaScript.
π AV News Stream: Revolutionizing News Consumption OO
AV (Audio-Visual) News Stream is the ultimate platform for streaming, reading, and listening to the latest news from diverse sources. Designed to transform your news consumption experience, it combines cutting-edge technology with a seamless user interface for unparalleled convenience.
This technical deep dive explores the architecture, implementation challenges, and solutions that power this innovative news platform.
π₯ [Video Demonstration]
π Links
- π Access the App: AV News Stream
- π» View the Code: GitHub Repository
The Problem Space
Modern news consumption faces three critical challenges:
- Limited Interaction: Traditional news platforms offer a passive, "read-only" experience that fails to engage users effectively.
- Reading Friction: The requirement for undivided attention during news consumption creates significant barriers:
- Disrupts daily activities
- Increases screen time
- Causes eye strain
- Limits multitasking capabilities
- Information Overload: Users struggle with:
- Overwhelming volume of news sources
- Difficulty in filtering relevant content
- Challenges in accessing real-time updates
- Limited ability to process information efficiently
The Solution
AV News Stream solves these challenges with:
1. Seamless Text-to-Speech Integration
Implemented a sophisticated voice integration system that:
- Utilizes Web Speech API for natural text-to-speech conversion
- Enables hands-free browsing experience
- Reduces screen time and eye strain
- Facilitates multitasking while consuming news
Implementation Example:
const textToSpeech = (text) => {
const speech = new SpeechSynthesisUtterance();
speech.text = text;
speech.rate = 1.0; // Configurable speech rate
speech.pitch = 1.0; // Natural pitch
speech.volume = 1.0;
window.speechSynthesis.speak(speech);
};
2. Real-Time Updates System
Integrated multiple APIs for comprehensive coverage:
- News API for diverse article sources
- Gnews.io for real-time updates
- YouTube API for live news streaming
- GitHub API for repository tracking
- Notion API for project documentation
Integration Example:
const fetchNewsData = async () => {
try {
const [NewsOrgArticles, GNewsArticles] = await Promise.all([
fetchNewsapiAPI(),
fetchGNewsAPI()
]);
// Merge and deduplicate articles
const mergedArticles = [...GNewsArticles, ...NewsOrgArticles]
.filter((article, index, self) =>
index === self.findIndex((t) => t.title === article.title)
);
return mergedArticles;
} catch (error) {
console.error('Error fetching news:', error);
throw error;
}
};
3. Enhanced User Interaction
Implemented advanced features for improved user experience:
- Conversational voice control
- Advanced search functionality
- Custom hooks for optimization
- Error handling system
- Email.js integration for communications
4. Comprehensive Coverage
Aggregates news from over 80,000 sources for global insights.
π οΈ Technical Architecture
Core Technology Stack
1. Frontend Framework
- React with Vite for optimized development
- JavaScript for core functionality
- PostCSS for advanced styling
2.State Management
- Redux Toolkit for global state
- React Context for component state
const saveSlice = createSlice({
name: "save",
initialState: {
savedArticles: [],
},
reducers: {
addArticle: (state, action) => {
const isArticleAlreadySaved = state.savedArticles.some(
article => article.title === action.payload.title
);
if (!isArticleAlreadySaved) {
state.savedArticles.push(action.payload);
}
}
}
});
3. Styling Solutions
- Tailwind CSS for utility-first styling
- DaisyUI for enhanced UI components
4. API Integration
- Multiple news APIs integration
- YouTube API for video content
- Email.js for contact functionality
5. Performance Optimization
- Lazy loading implementation
- Shimmer UI for loading states
- Custom hooks for efficient data fetching
π‘ Key Features
1. Voice-Enabled News Reading
The standout feature is voice integration using the Web Speech API. Users can enjoy a hands-free experience, making it easier to stay informed while multitasking.
Hereβs how the text-to-speech functionality is implemented:
const textToSpeech = (text) => {
const speech = new SpeechSynthesisUtterance();
speech.text = text;
window.speechSynthesis.speak(speech);
};
2. Article Save Functionality
Powered by Redux Toolkit, users can save their favorite articles for later viewing, ensuring an organized and personalized experience:
const saveSlice = createSlice({
name: "save",
initialState: {
savedArticles: [],
},
reducers: {
addArticle: (state, action) => {
const isArticleAlreadySaved = state.savedArticles.some(
article => article.title === action.payload.title
);
if (!isArticleAlreadySaved) {
state.savedArticles.push(action.payload);
}
},
removeArticle: (state, action) => {
state.savedArticles = state.savedArticles.filter(
article => article.title !== action.payload.title
);
}
}
});
3. Integration of Multiple APIs
- π° News API: Access a diverse range of news articles, including archived content.
- π Gnews.io: Stay updated with breaking news and trending topics.
- πΉ YouTube API: Stream live news videos for real-time updates.
- π GitHub API: Track changes in repositories.
- π Notion API: Add project descriptions through Notion pages.
4. Advanced Search Functionality
The search feature has been optimized to reduce excessive API calls and enhance efficiency:
const handleSearch = async () => {
if (searchText) {
try {
const queryUrl = `/api/search?query=${encodeURIComponent(searchText)}`;
const response = await fetch(queryUrl);
const data = await response.json();
setNewsCopy(data.articles || []);
} catch (error) {
console.error('Error fetching search results:', error);
setNewsCopy([]);
}
}
};
π§ Technical Challenges & Solutions
1. CORS Implementation
Challenge: NewsAPI.org's free tier limitations in production.
Solution: Implemented a custom Express backend:
import express from 'express';
import cors from 'cors';
const app = express();
app.use(cors());
app.get('/api/news', async (req, res) => {
const { category } = req.query;
try {
let url = `https://newsapi.org/v2/top-headlines?country=${country}&apiKey=${API_KEY}`;
const response = await fetch(url);
const data = await response.json();
res.json(data);
} catch (error) {
res.status(500).json({ error: 'Failed to fetch news' });
}
});
2. Data Quality Management
Challenge: Inconsistent data from multiple APIs.
Solution: Integrated multiple sources with robust validation:
const fetchNewsData = async () => {
const [NewsOrgArticles, GNewsArticles] = await Promise.all([
fetchNewsapiAPI(),
fetchGNewsAPI()
]);
const mergedArticles = [...GNewsArticles, ...NewsOrgArticles];
return mergedArticles;
};
3. Search Optimization
Challenge: API exhaustion due to frequent calls.
Solution: Search implementation refactored to reduce API hits (as shown above).
4. Null Data Handling
Challenge: Inconsistent data from multiple APIs.
Solution: Implemented robust validation:
const validateArticle = (article) => {
return {
...article,
title: article?.title || 'Untitled',
description: article?.description || 'No description available',
imageUrl: article?.imageUrl || defaultImage,
author: article?.author || 'Unknown Author'
};
};
const processArticles = (articles) => {
return articles
.filter(article => article.title && article.description)
.map(validateArticle);
};
πAdvantages
1. Accessibility Features
- Hands-free voice assistant integration.
- Text-to-speech functionality for multitasking.
2. Performance Optimizations
- Lazy loading for faster page loads.
- Shimmer UI for a smooth visual experience.
3. Real-Time Updates
- Aggregates content from multiple APIs for the latest news.
- Includes live news streaming functionality.
π«Current Limitations
1. API Constraints
- Limited requests on free-tier APIs.
- Rate-limiting affects high-volume use.
2. Feature Gaps
- No user authentication system.
- No offline functionality.
3. Dependencies
- Requires stable internet connectivity.
- Relies on browser compatibility for voice features.
π οΈ Future Roadmap
User Authentication
- Personal news preferences.
- Syncing saved articles.
- Custom reading lists.
Offline Capabilities
- Article caching.
- Background sync for offline reading.
Advanced Features
- AI-powered content recommendations.
- Enhanced voice commands.
- Social sharing integration.
π―Technical Learnings
The development of AV News Stream provided valuable insights into:
API Integration
- Managing multiple data sources.
- Effective error handling and rate limiting.
Performance Optimization
- Lazy loading implementation.
- Efficient state management practices.
Voice Integration
- Implementing the Web Speech API.
- Processing voice commands effectively.
Let's Create Something Amazing Together!
Whether you have a project in mind or just want to connect, I'm always excited to collaborate and bring ideas to life.
Continue the Journey
Thanks for taking the time to explore my work! Let's connect and create something amazing together.